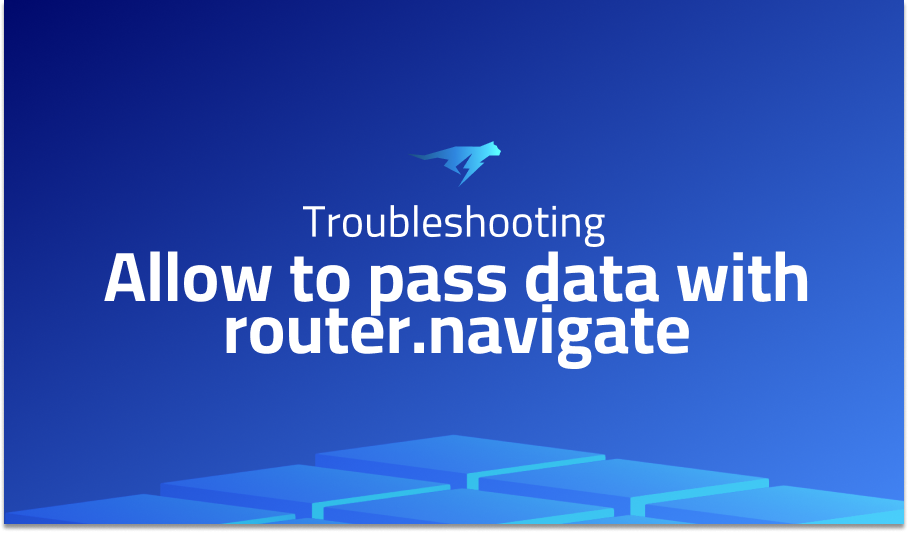
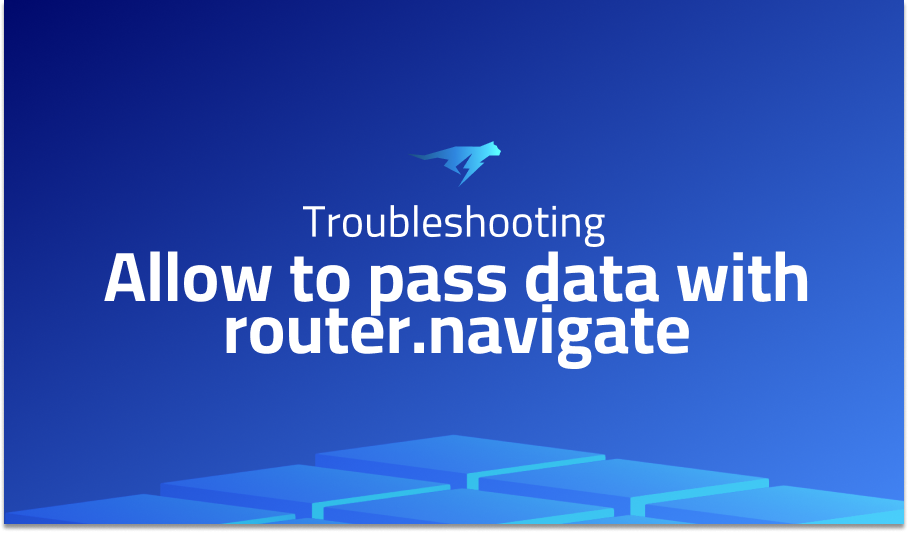
Allow to pass data with router.navigate
Explanation of the problem
The submission is a feature request to address a current limitation in Angular. The issue at hand is the absence of a built-in mechanism in Angular to pass additional data when using the router.navigate
method. Several related issues have been raised in the Angular repository, but unfortunately, they were closed without being resolved. These issues, such as #5217, #8515, #9804, and #10248, highlight the need for the ability to pass data along with navigation.
The expected behavior, as proposed in #5217, suggests extending the router.navigateByUrl
method to accept an additional parameter for data:
this.router.navigateByUrl('/path', { data: { entity: 'entity' } });
With this enhancement, the provided data should be accessible across various parts of the application where route.data
is available, including the target component and any associated resolvers.
The issue affects Angular versions 2 and above. While a workaround in the form of a library called Angular2-navigate-with-data has been suggested by user @Hipparch, it would be beneficial to have a native solution integrated into Angular to address this limitation effectively.
Troubleshooting with the Lightrun Developer Observability Platform
Getting a sense of what’s actually happening inside a live application is a frustrating experience, one that relies mostly on querying and observing whatever logs were written during development.
Lightrun is a Developer Observability Platform, allowing developers to add telemetry to live applications in real-time, on-demand, and right from the IDE.
- Instantly add logs to, set metrics in, and take snapshots of live applications
- Insights delivered straight to your IDE or CLI
- Works where you do: dev, QA, staging, CI/CD, and production
Start for free today
Problem solution for: Allow to pass data with router.navigate
To address the issue of passing additional data with router.navigate
in Angular, one possible solution is to leverage query parameters to carry the required information. This approach allows us to append data to the URL and extract it in the target component or resolver.
Here’s an example of how you can implement this solution:
- When navigating, encode the additional data as query parameters:
const data = { entity: 'entity' };
const queryParams = new URLSearchParams(data).toString();
this.router.navigate(['/path'], { queryParams });
- In the target component, extract the data from the query parameters:
import { ActivatedRoute } from '@angular/router';
constructor(private route: ActivatedRoute) {}
ngOnInit() {
this.route.queryParams.subscribe(params => {
const data = params as any; // Cast the params to the desired data type
// Use the extracted data as needed
console.log(data.entity);
});
}
By encoding the data as query parameters and extracting them in the target component, you can achieve the desired functionality of passing additional information during navigation in Angular.
It’s important to note that this solution requires appropriate encoding and decoding of data to handle special characters or complex objects. Additionally, consider the security implications when passing sensitive information via query parameters and ensure proper validation and sanitization.
Problems with angular
- Problem: “Cannot read property ‘length’ of undefined” error when using the
async
pipe with aBehaviorSubject
. Description: Users have reported an error when using theasync
pipe with aBehaviorSubject
. The error message is “Cannot read property ‘length’ of undefined”. This error occurs when theBehaviorSubject
has not yet emitted any values and theasync
pipe tries to access thelength
property of the value. Solution: One solution is to initialize theBehaviorSubject
with a default value. For example:
private dataSubject = new BehaviorSubject<any[]>([]);
data$ = this.dataSubject.asObservable();
Then, in the component, check if the value is undefined before accessing its length property:
<ng-container *ngIf="data$ | async as data">
<div *ngIf="data.length > 0">
// Display data here
</div>
</ng-container>
- Problem: Error “zone.js has detected that ZoneAwarePromise
(window|global).Promise
has been overwritten” when running Angular tests. Description: When running Angular tests, users have reported an error message that says “zone.js has detected that ZoneAwarePromise(window|global).Promise
has been overwritten”. This error occurs when a third-party library, such as a polyfill, overwrites the globalPromise
object. Solution: One solution is to use thezone.js
library to patch the globalPromise
object before running the tests. Add the following line to thetest.ts
file:
import 'zone.js/testing';
Then, in the beforeEach
function of the test, patch the Promise
object:
beforeEach(() => {
(window as any)['Promise'] = Promise;
});
- Problem: Error “Maximum call stack size exceeded” when using
ng serve
. Description: Some users have reported an error message that says “Maximum call stack size exceeded” when running theng serve
command. This error occurs when there is a circular dependency in the application. Solution: One solution is to use the Angular dependency injection system to break the circular dependency. For example, ifComponentA
depends onComponentB
andComponentB
depends onComponentA
, create a new service and move the shared logic to the service. Then, inject the service into both components. For example:
@Injectable({ providedIn: 'root' })
export class SharedService {
// Shared logic here
}
@Component({...})
export class ComponentA {
constructor(private sharedService: SharedService) {}
// Use the shared service here
}
@Component({...})
export class ComponentB {
constructor(private sharedService: SharedService) {}
// Use the shared service here
}
A brief introduction to angular
Angular is a popular open-source web application framework used to build dynamic single-page applications. The Angular framework is written in TypeScript, a superset of JavaScript that allows for static typing and other features. The primary goal of Angular is to provide a robust and efficient framework for building web applications that can be easily maintained and scaled. Angular is known for its powerful features such as two-way data binding, dependency injection, and an extensive library of built-in directives and services.
One of the main benefits of Angular is its component-based architecture, where each component represents a specific feature or view in the application. Components are reusable and can be composed to create complex UIs. Angular also supports reactive programming and provides a robust set of APIs for working with observables, which allows for easier handling of asynchronous operations. The framework has a steep learning curve, but once mastered, developers can build complex and scalable web applications in a relatively short time. Overall, Angular is a versatile framework that offers a lot of power and flexibility to developers.
Most popular use cases for angular
-
- Building dynamic and scalable web applications: Angular provides a comprehensive framework for building modern web applications that are scalable, maintainable, and easy to develop. It offers a wide range of tools and features that make it easy to create dynamic and interactive web applications that can handle large amounts of data and complex workflows.
- Creating reusable UI components: Angular’s component-based architecture allows developers to create reusable UI components that can be easily integrated into different parts of an application. This feature makes it easier to maintain and update applications over time, as changes made to a component are automatically propagated to all instances of that component in the application.
Here’s an example code block for creating a reusable component in Angular:
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-button',
template: `
<button [class]="classes">
{{label}}
</button>
`
})
export class ButtonComponent {
@Input() label: string;
@Input() classes: string;
}
- Developing cross-platform applications: With Angular, developers can build applications that can run on multiple platforms, including web, mobile, and desktop. This is made possible by using technologies such as Angular Universal for server-side rendering, and frameworks like Ionic for mobile app development. By leveraging these tools, developers can create a single codebase that can be used to build applications for multiple platforms.
Here’s an example code block for building a cross-platform application with Angular and Ionic:
# Install Ionic CLI
npm install -g @ionic/cli
# Create a new Ionic app with Angular
ionic start myApp --type=angular
# Add a new page to the app
ionic generate page myPage
# Build and run the app on a mobile device
ionic capacitor add ios
ionic capacitor copy ios
ionic capacitor open ios
It’s Really not that Complicated.
You can actually understand what’s going on inside your live applications.