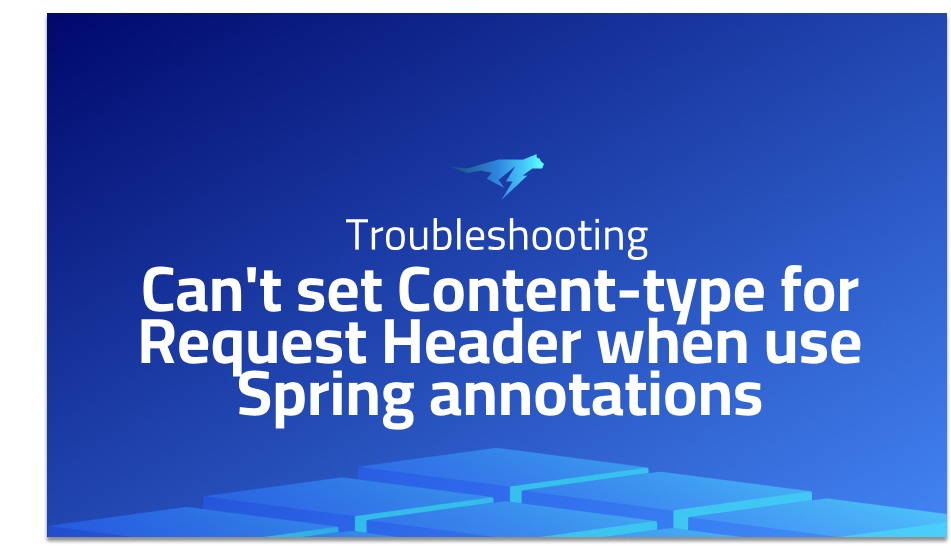
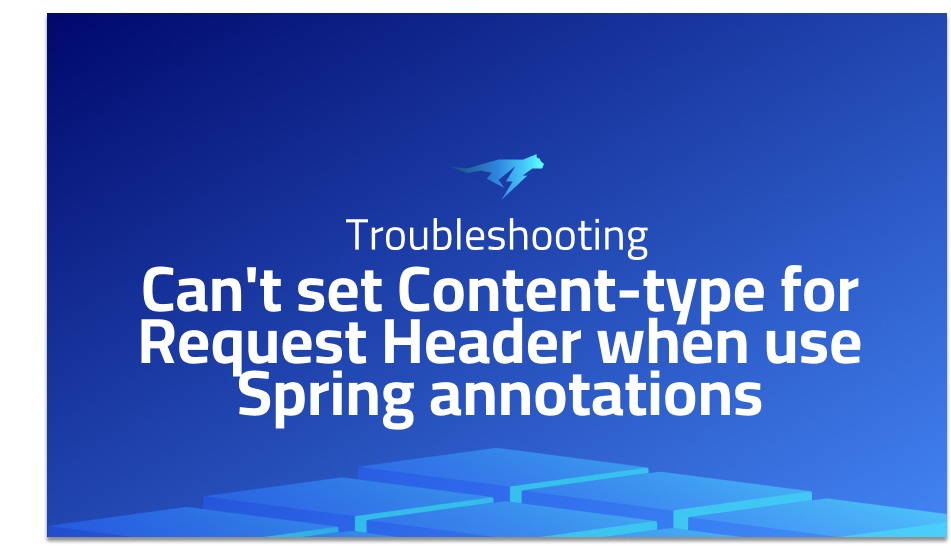
Can’t set Content-type for Request Header when use Spring annotations
Explanation of the problem
When using Feign, a declarative web service client, in combination with Spring annotations, a problem arises when trying to specify the “produces” value for a request that expects to accept “text/plain” content. In the following code snippet, the @PostMapping annotation is used to define an endpoint “/demo” that consumes “application/json” and produces “text/plain”:
@PostMapping(value = "/demo", consumes = "application/json", produces = "text/plain")
public String demo(@RequestBody List<Long> ids) {
// Method implementation
}
However, despite explicitly setting the “produces” value to “text/plain”, the request header “Accept” still contains “application/json”. This behavior indicates that the “produces” value is not being applied correctly. As a result, the server may not respond with the expected “text/plain” content type, leading to unexpected behavior or errors.
Troubleshooting with the Lightrun Developer Observability Platform
Getting a sense of what’s actually happening inside a live application is a frustrating experience, one that relies mostly on querying and observing whatever logs were written during development.
Lightrun is a Developer Observability Platform, allowing developers to add telemetry to live applications in real-time, on-demand, and right from the IDE.
- Instantly add logs to, set metrics in, and take snapshots of live applications
- Insights delivered straight to your IDE or CLI
- Works where you do: dev, QA, staging, CI/CD, and production
Start for free today
Problem solution for: Can’t set Content-type for Request Header when use Spring annotations
To address this issue, it is necessary to ensure that the “produces” value is correctly applied to the Feign client and the server-side endpoint. One potential solution is to modify the Feign client configuration to explicitly set the “Accept” header value to “text/plain” for the corresponding request. The following code snippet demonstrates how this can be achieved:
@FeignClient(name = "demoClient", url = "http://example.com", configuration = DemoClientConfiguration.class)
public interface DemoClient {
@PostMapping(value = "/demo", consumes = "application/json", produces = "text/plain")
@Headers("Accept: text/plain")
String demo(@RequestBody List<Long> ids);
}
By explicitly specifying the “Accept” header value using the @Headers annotation, the Feign client will send the correct “Accept” header to the server, indicating that it expects “text/plain” content in the response. This helps ensure that the server processes the request according to the desired content type.
Other popular problems with feign
Problem 1: One common issue encountered when using Feign, a declarative web service client, is handling error responses returned by the server. By default, Feign treats any non-2xx HTTP response code as an error and throws a FeignException. However, the exception may not provide sufficient information about the specific error that occurred, making it challenging to handle and process the error appropriately.
@FeignClient(name = "exampleClient", url = "http://example.com")
public interface ExampleClient {
@GetMapping("/api/resource")
String getResource();
}
To address this problem, you can customize the Feign error decoder to extract relevant information from the error response and provide a more meaningful exception. This can be achieved by implementing the ErrorDecoder interface and overriding the decode() method. Here’s an example of a custom error decoder that extracts the error message from the response body:
public class CustomErrorDecoder implements ErrorDecoder {
private final ErrorDecoder defaultErrorDecoder = new Default();
@Override
public Exception decode(String methodKey, Response response) {
if (response.status() == HttpStatus.BAD_REQUEST.value()) {
String errorMessage = extractErrorMessage(response.body());
return new CustomException(errorMessage);
}
// Delegate to the default error decoder for other error codes
return defaultErrorDecoder.decode(methodKey, response);
}
private String extractErrorMessage(Response.Body body) {
// Extract the error message from the response body
// Implement your logic here
}
}
By implementing a custom error decoder, you can extract specific details from the error response and throw a custom exception that encapsulates the relevant information. This allows for more effective error handling and troubleshooting in your Feign client.
Problem 2: Another common problem with Feign is dealing with complex request payloads or handling dynamic parameters in the request. Feign provides support for sending GET, POST, PUT, and DELETE requests, but it may not be immediately obvious how to pass complex data structures or dynamically construct the request URL.
@FeignClient(name = "exampleClient", url = "http://example.com")
public interface ExampleClient {
@PostMapping("/api/resource")
void createResource(ResourceRequest request);
}
One solution to this problem is to leverage Feign’s support for parameter annotations, such as @RequestBody and @RequestParam. These annotations allow you to specify the request payload and query parameters in a more structured manner. Here’s an example:
@FeignClient(name = "exampleClient", url = "http://example.com")
public interface ExampleClient {
@PostMapping("/api/resource")
void createResource(@RequestBody ResourceRequest request, @RequestParam("param") String param);
}
In this example, the @RequestBody annotation is used to pass the request payload, while the @RequestParam annotation is used to include a query parameter in the request URL. By using these annotations appropriately, you can handle complex request payloads and dynamic parameters in a more structured and maintainable way.
Problem 3: Feign may encounter issues with long request URLs or parameters exceeding the URL length limit imposed by the server or the HTTP specification. This can result in HTTP 414 (URI Too Long) errors or incomplete requests.
To overcome this problem, you can configure Feign to use HTTP POST with a request body instead of GET for requests with long URLs or large parameters. This allows you to send the data in the request body instead of appending it to the URL.
@FeignClient(name = "exampleClient", url = "http://example.com")
public interface ExampleClient {
@RequestMapping(value = "/api/resource", method = RequestMethod.POST)
void getResource(@RequestParam("param") String param, @RequestBody LargeRequestBody requestBody);
}
By using POST instead of GET and passing the large data in the request body, you can avoid the URL length limitation and ensure the successful transmission of the request to the server.
These are just a few examples of common problems encountered when using Feign and potential solutions to address them. By understanding these challenges and implementing appropriate solutions, you can enhance the reliability and effectiveness of your Feign-based web service clients.
A brief introduction to feign
Feign is a declarative web service client developed by Netflix that simplifies the integration of RESTful APIs into Java applications. It provides a higher-level, annotation-based approach for defining and consuming HTTP-based services, allowing developers to focus on the functionality of their applications rather than dealing with low-level HTTP client code. With Feign, developers can easily make HTTP requests to remote services and map the responses to Java objects, reducing the boilerplate code typically associated with manual HTTP communication.
Feign leverages the power of annotations to define the API contract and configuration details. By using annotations such as @FeignClient, @GetMapping, @PostMapping, and others, developers can express the desired API endpoints and HTTP methods in a concise and intuitive manner. Feign also integrates seamlessly with other popular libraries in the Java ecosystem, such as Spring Cloud and Spring Boot, enabling the creation of robust and scalable microservices architectures. Additionally, Feign supports advanced features like request and response interception, error handling, and request retries, providing developers with flexible and extensible options for building resilient and reliable web service clients.
In summary, Feign offers a convenient and efficient way to consume RESTful APIs in Java applications. Its declarative and annotation-based approach reduces the complexity of working with HTTP requests and responses, allowing developers to focus on business logic and application functionality. With its integration capabilities and support for advanced features, Feign is a powerful tool for building robust and scalable microservices architectures in the Java ecosystem.
Most popular use cases feign
- Service Integration: Feign can be used to integrate and consume RESTful services in Java applications. By defining interfaces with appropriate annotations, developers can easily interact with remote APIs and perform HTTP operations such as GET, POST, PUT, and DELETE. Feign abstracts away the complexities of low-level HTTP client code and provides a higher-level abstraction for making service calls. Here’s an example of how Feign interface can be defined to interact with a RESTful API:
@FeignClient(name = "myApiClient", url = "https://api.example.com")
public interface MyApiClient {
@GetMapping("/users/{id}")
User getUserById(@PathVariable("id") Long id);
@PostMapping("/users")
User createUser(@RequestBody User user);
}
- Load Balancing and Service Discovery: Feign integrates seamlessly with service discovery mechanisms and load balancing libraries, making it suitable for building scalable and resilient microservices architectures. By leveraging technologies like Spring Cloud and Netflix Eureka, Feign can automatically discover available service instances and distribute requests across them. This allows developers to build distributed systems that can dynamically adapt to changes in the service topology. Feign’s integration with load balancing libraries like Ribbon provides additional flexibility in load distribution. Here’s an example of using Feign with Spring Cloud and Eureka:
@FeignClient(name = "myService", fallback = MyServiceFallback.class)
@RibbonClient(name = "myService")
public interface MyServiceClient {
@GetMapping("/api/data")
String getData();
}
- Advanced Features and Extensibility: Feign offers advanced features and extensibility options to cater to various use cases. It supports features like request and response interception, allowing developers to customize the behavior of service calls. Feign also provides error handling mechanisms, enabling graceful handling of exceptions and error responses from the remote service. Developers can extend Feign’s functionality by implementing custom interceptors, error handlers, and decoders. This level of flexibility empowers developers to adapt Feign to their specific requirements and integrate it seamlessly into their application architecture.
It’s Really not that Complicated.
You can actually understand what’s going on inside your live applications.