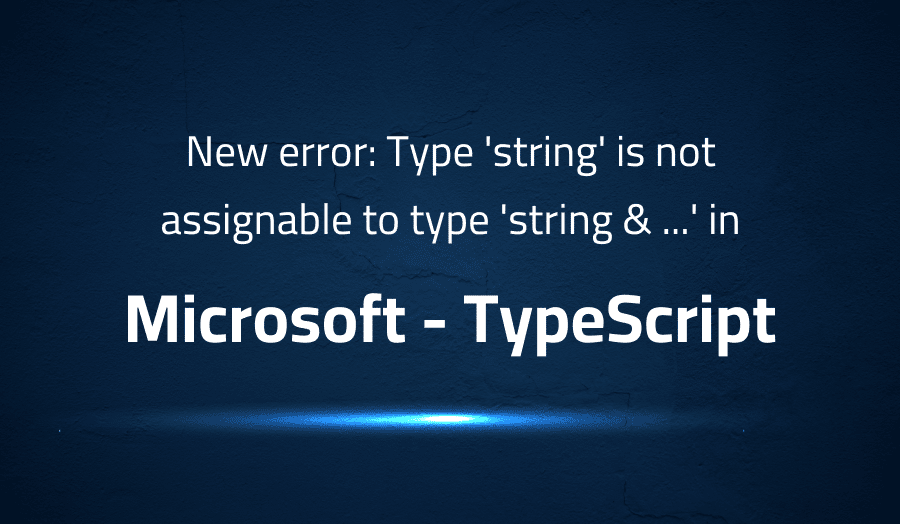
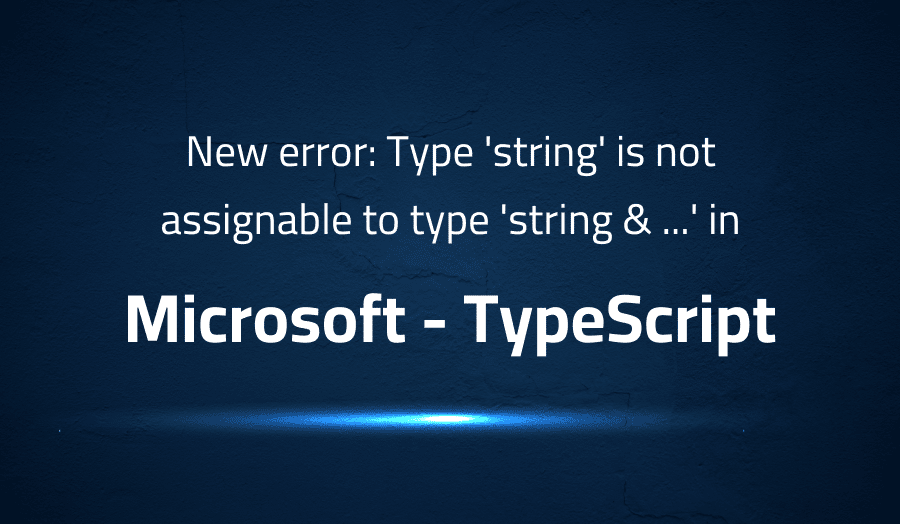
New error: Type ‘string’ is not assignable to type ‘string & …’ in Microsoft TypeScript
Explanation of the problem
The error message in the problem description is indicating that there is an issue with the type of the variable being assigned to the style property on lines 109 and 119 of the mwc-linear-progress-base.ts file. The error is specifically that the type of the variable being assigned is ‘string’, but it should be of a type that includes a function that takes a property of type ‘string’ and returns a value of type ‘string’.
Here is the relevant code block that is causing the error:
109 this.bufferElement
.style[property as Exclude<keyof CSSStyleDeclaration, 'length'|'parentRule'>] =
119 this.primaryBar
.style[property as Exclude<keyof CSSStyleDeclaration, 'length'|'parentRule'>] =
The ‘Exclude<keyof CSSStyleDeclaration, ‘length’|’parentRule’>’ is used here to narrow down the type of the property to exclude ‘length’ and ‘parentRule’ from the set of possible values.
To fix this issue, the variable being assigned to the style property should be changed to a type that includes the required function for the assignment to be valid. This could be done by using a type that explicitly defines the function, or by using a type that is known to include the function such as the ‘CSSStyleDeclaration’ type.
In order to reproduce this error, the following steps should be taken:
- Run
npm ci
to install the necessary dependencies - Run
npm run build:styling
to build the styling - Run
tsc -b -f
to transpile the TypeScript code
The error will occur during the transpilation step as the code will fail to meet the expected type.
Troubleshooting with the Lightrun Developer Observability Platform
Getting a sense of what’s actually happening inside a live application is a frustrating experience, one that relies mostly on querying and observing whatever logs were written during development.
Lightrun is a Developer Observability Platform, allowing developers to add telemetry to live applications in real-time, on-demand, and right from the IDE.
- Instantly add logs to, set metrics in, and take snapshots of live applications
- Insights delivered straight to your IDE or CLI
- Works where you do: dev, QA, staging, CI/CD, and production
Start for free today
Problem solution for New error: Type ‘string’ is not assignable to type ‘string & …’ in Microsoft TypeScript
The error message “Type ‘string’ is not assignable to type ‘string & …'” is indicating that the variable being assigned is of type ‘string’, but the type it is being assigned to is expecting a more complex type that includes a string but also has additional properties or methods.
The specific type that is being referenced in the error message ‘string & ((property: string) => string) & ((property: string) => string) & ((index: number) => string) & ((property: string) => string) & ((property: string, value: string | null, priority?: string | undefined) => void)’ is a combination of several types using the & (and) operator. This type is essentially saying that the variable being assigned should be a string and also have the properties/methods of several other types such as function that takes a property and returns a string, function that takes an index and returns a string and function that takes a property and a value and returns void.
This error is typically caused by passing a variable of the wrong type to a function or property that is expecting a more complex type. In this case, the variable being assigned to the ‘style’ property is a string, but it is expecting a type that has additional properties/methods.
To fix this error, the variable being assigned should be changed to a type that includes the additional properties/methods as required by the type it is being assigned to. This can be done by using the correct type for the variable or by casting the variable to the correct type.
Here is an example of how casting the variable to the correct type:
this.bufferElement.style[property as Exclude<keyof CSSStyleDeclaration, 'length'|'parentRule'>] = value as CSSStyleDeclaration[Exclude<keyof CSSStyleDeclaration, 'length'|'parentRule'>];
In this example, the variable value is cast to the correct type using the ‘as’ keyword.
It’s also important to note that this error can also be caused by a mismatch in the version of typescript or other dependencies, or even a missing or outdated types declarations.
Other popular problems with TypeScript
Problem: Type ‘any’ is not assignable to type ‘X’:
This error occurs when a variable of type ‘any’ is being assigned to a variable of a specific type ‘X’. TypeScript is designed to catch type mismatches at compile time to prevent runtime errors, but when a variable is of type ‘any’, TypeScript cannot infer its type and will allow any type of value to be assigned to it.
Here is an example of how this error might occur:
function doSomething(value: any) {
let x: string = value;
}
In this example, the function ‘doSomething’ takes in a parameter of type ‘any’ and then assigns it to a variable of type ‘string’. Since the value passed in could be of any type, TypeScript will raise an error because it cannot guarantee that the value is a valid string.
Solution:
To fix this error, the type of the variable should be explicitly set or inferred by the compiler. One way to achieve this is by using a type assertion:
function doSomething(value: any) {
let x: string = value as string;
}
Alternatively, the function parameter type can be set to the expected type:
function doSomething(value: string) {
let x: string = value;
}
Problem: Property ‘X’ does not exist on type ‘Y’:
This error occurs when trying to access a property that does not exist on a given type. This can happen when working with complex types such as interfaces or classes, or when using a third-party library that has its own types.
Here is an example of how this error might occur:
interface MyObject {
name: string;
age: number;
}
let obj: MyObject = {name: 'John', age: 30};
console.log(obj.address);
In this example, the code is trying to access the ‘address’ property on an object that only has ‘name’ and ‘age’ properties. TypeScript will raise an error because it cannot find a property called ‘address’ on the type ‘MyObject’.
Solution:
To fix this error, the code should be updated to access only the properties that exist on the type. One way to achieve this is by using a type guard:
if ('address' in obj) {
console.log(obj.address);
}
Alternatively, the code can be updated to only access properties that are known to exist on the type:
console.log(obj.name);
console.log(obj.age);
Problem: Argument of type ‘X’ is not assignable to parameter of type ‘Y’:
This error occurs when a function or method is called with an argument of a type that does not match the type of the parameter it is being passed to. This can happen when working with complex types such as interfaces or classes, or when using a third-party library that has its own types.
Here is an example of how this error might occur:
function doSomething(value: number) {
console.log(value);
}
doSomething('hello');
In this example, the function ‘doSomething’ takes in a parameter of type ‘number’, but it is being called with an argument of type ‘string’. TypeScript will raise an error because it cannot guarantee that the ‘hello’ is a valid number.
Solution:
To fix this error, the argument being passed to the function should be of the correct type. One way to achieve this is by using a type assertion:
doSomething(Number('hello') as number);
Alternatively, the argument can be converted to the correct type before being passed to the function:
let numValue = parseInt('hello');
doSomething(numValue);
Another way is to overload the function with different types of parameters.
function doSomething(value: number): void;
function doSomething(value: string): void;
function doSomething(value: number | string): void {
if (typeof value === 'number') {
console.log(value);
} else {
console.log(Number(value));
}
}
In this example, the function is overloaded to accept both ‘number’ and ‘string’ types as valid parameters and based on the type of value passed, it will call the corresponding function.
A brief introduction to TypeScript
TypeScript is a programming language that is a strict syntactical superset of JavaScript. It adds optional static typing and other features such as classes, interfaces, and modules to JavaScript. TypeScript allows developers to catch errors at compile-time, rather than at runtime, and also provides better tooling support.
TypeScript is particularly useful when working on large, complex codebases that have many developers working on them simultaneously. By adding a type system to JavaScript, TypeScript makes it easier to catch errors early and to understand the shape of the data being passed around in the application. Additionally, TypeScript’s support for interfaces and classes allows for better code organization and reuse. The type information is used by the TypeScript compiler to check for type correctness and generate JavaScript that runs on any browser or host. This makes it an ideal choice for applications that need to run on multiple platforms and environments.
TypeScript also provides a way to annotate existing JavaScript code with types and make it more robust and self-documenting. The type annotations are optional, so developers can start using TypeScript incrementally, by adding types to their JavaScript code as needed. This allows developers to introduce TypeScript gradually into existing codebases, and to adopt it as they become more comfortable with the language.
Most popular use cases for TypeScript
- TypeScript can be used for adding optional static typing to JavaScript, which allows for better tooling support, improved code readability and maintainability, and catching errors at compile-time instead of runtime. This allows for more robust and scalable codebases as the application grows in complexity and size.
- TypeScript can be used to implement object-oriented programming concepts such as classes and interfaces, which allows for better code organization and reuse. This is particularly useful when working on large, complex codebases that have many developers working on them simultaneously.
- TypeScript can be used to create definition files for external JavaScript libraries, which allows for better code editor support and improved development experience. Definition files provide type information for libraries and modules, which allows the TypeScript compiler to check for type correctness and provide better code completion and error checking.
Here is an example of how to create a definition file for an external JavaScript library:
// example.d.ts
declare module "my-library" {
export function myFunction(arg: string): number;
export class MyClass {
constructor(arg: string);
public myMethod(): void;
}
}
This example defines a module “my-library” which includes a function and a class with a constructor and a method. By including this file in your TypeScript project, you can use the library with full type checking and code completion.
It’s Really not that Complicated.
You can actually understand what’s going on inside your live applications.