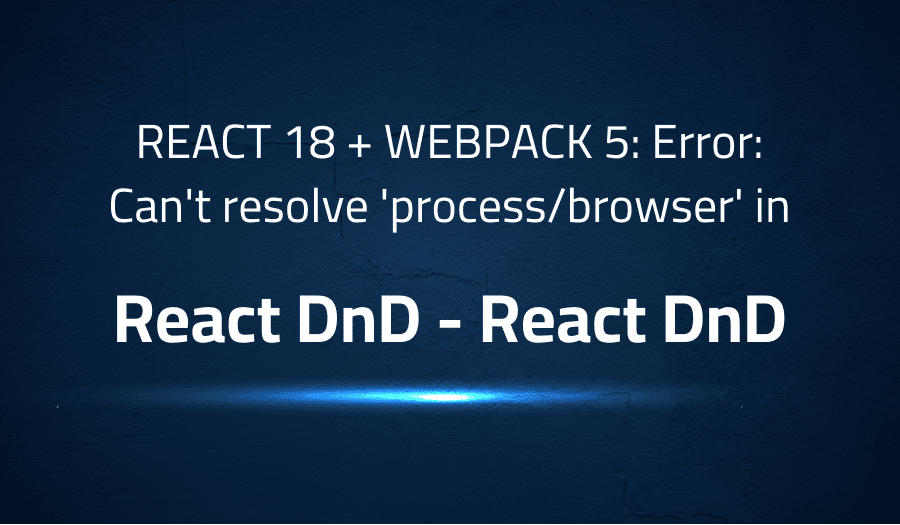
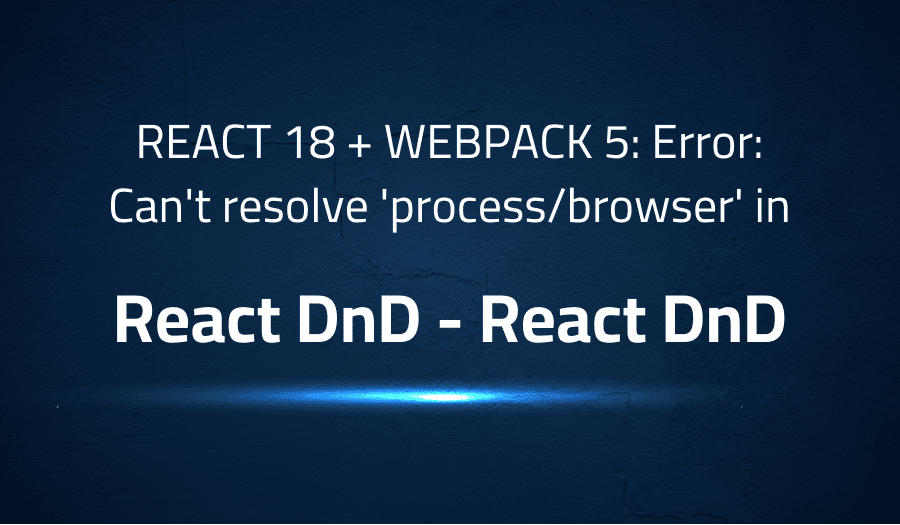
REACT 18 + WEBPACK 5: Error: Can’t resolve ‘process/browser’ in React DnD React DnD
Explanation of the problem
The current issue has been identified in the “./node_modules/@react-dnd/invariant/dist/index.js” file, at line 42, column 16 to 23. The error message displays “Module not found: Error: Can’t resolve ‘process/browser’ in ‘/Users/tommix/DEVELOPMENT/ARES/ares-ui/ares-control-center/node_modules/@react-dnd/invariant/dist’. Did you mean ‘browser.js’?” This error has been caused by the fully specified request of ‘process/browser’ failing to resolve.
To reproduce this issue, the following steps can be followed:
mpx create-react-app
npm install react-dnd
In the “/src/index.js” file, add the following code:
import { useDrag } from "react-dnd";
After running “npm run”, the expected behavior is for there to be no errors in the compilation process, without any need for changing the webpack config.
As a workaround for this issue, the following steps can be taken:
npm install react-app-rewired -d
Then, create a “./config-overrides.js” file and add the following code:
config.module.rules.unshift({
test: /\.m?js$/,
resolve: {
fullySpecified: false, // disable the behavior
},
});
Troubleshooting with the Lightrun Developer Observability Platform
Getting a sense of what’s actually happening inside a live application is a frustrating experience, one that relies mostly on querying and observing whatever logs were written during development.
Lightrun is a Developer Observability Platform, allowing developers to add telemetry to live applications in real-time, on-demand, and right from the IDE.
- Instantly add logs to, set metrics in, and take snapshots of live applications
- Insights delivered straight to your IDE or CLI
- Works where you do: dev, QA, staging, CI/CD, and production
Start for free today
Problem solution for REACT 18 + WEBPACK 5: Error: Can’t resolve ‘process/browser’ in React DnD React DnD
The error message “Module not found: Error: Can’t resolve ‘process/browser'” is a common issue when using the react-dnd library. It occurs due to a missing module in the “@react-dnd/invariant/dist/index.js” file, which requires the “process/browser” module. This error can prevent the compilation process from running smoothly, making it necessary to resolve it.
Solution to the issue:
By installing the “process” module via npm, you can then add a fallback in the webpack config to resolve the “process/browser” module. This is done by adding the following code block to your webpack config:
resolve: {
fallback: {
'process/browser': require.resolve('process/browser'),
}
}
This code block specifies the location of the “process/browser” module, and allows the webpack config to resolve it properly.
There is also a workaround that requires modifying the webpack config if you used create-react-app. To do this, you would need to use the react-app-rewired package. The proposed solution involves adding a resolve alias for the “react/jsx-runtime.js” and “react/jsx-dev-runtime.js” modules, as well as adding a module rule with fully specified set to false. This is done by adding the following code block to your webpack config:
{
resolve: {
alias: {
"react/jsx-dev-runtime.js": "react/jsx-dev-runtime",
"react/jsx-runtime.js": "react/jsx-runtime",
},
},
module: {
rules: [
{
test: /\.m?js$/,
resolve: {
fullySpecified: false
},
},
]
}
}
This code block not only resolves the “process/browser” issue but also the “jsx-runtime” issue, which is commonly encountered in similar issues.
Other popular problems with React DnD
Problem: “jsx-runtime” Error
Another common issue with react-dnd is the “jsx-runtime” error. This error occurs when the webpack config is unable to resolve the “react/jsx-runtime.js” and “react/jsx-dev-runtime.js” modules.
Solution:
The issue can be resolved by adding a resolve alias for these modules in the webpack config. The solution also involves adding a module rule with fully specified set to false. This is done by adding the following code block to your webpack config:
{
resolve: {
alias: {
"react/jsx-dev-runtime.js": "react/jsx-dev-runtime",
"react/jsx-runtime.js": "react/jsx-runtime",
},
},
module: {
rules: [
{
test: /\.m?js$/,
resolve: {
fullySpecified: false
},
},
]
}
}
Problem: React DnD requires the DragDropContext to be rendered at the top of your component tree” Error
This error occurs when the DragDropContext component is not properly rendered at the top of the component tree. The DragDropContext component provides the context for the react-dnd library, and it must be rendered at the top of the component tree in order for it to work properly.
Solution:
Make sure that the DragDropContext component is properly rendered at the top of the component tree. An example of the correct implementation is shown below:
import React from "react";
import { DragDropContext } from "react-dnd";
import HTML5Backend from "react-dnd-html5-backend";
import MyComponent from "./MyComponent";
const App = () => {
return (
<DragDropContext backend={HTML5Backend}>
<MyComponent />
</DragDropContext>
);
};
export default App;
In this example, the DragDropContext component is properly rendered at the top of the component tree, providing the context for the react-dnd library. The HTML5Backend is also specified as the backend for the context.
Problem: Error with “Could not find the drag source or drop target on the DOM”
This error message can occur when using React DnD, and it means that the drag source or drop target elements could not be found in the DOM, either because they have been unmounted or because they are not part of the render tree. This can happen when the DragSource or DropTarget higher-order components are used incorrectly or when there is an unexpected change in the component’s state or props.
Solution:
To resolve this issue, you should ensure that the DragSource and DropTarget components are correctly connected to the store and that they are properly configured with the correct collect and spec functions. You should also ensure that the elements are part of the render tree and not unmounted or hidden by some other means.
import { DragSource, DropTarget } from 'react-dnd';
const dragSourceSpec = {
beginDrag(props) {
return {};
}
};
const dragSourceCollect = (connect, monitor) => ({
connectDragSource: connect.dragSource(),
isDragging: monitor.isDragging()
});
const dropTargetSpec = {
drop(props, monitor) {
// Implement your logic here
}
};
const dropTargetCollect = (connect, monitor) => ({
connectDropTarget: connect.dropTarget(),
isOver: monitor.isOver(),
canDrop: monitor.canDrop()
});
class MyComponent extends React.Component {
render() {
const { connectDragSource, isDragging, connectDropTarget, isOver, canDrop } = this.props;
return connectDropTarget(
connectDragSource(
<div style={{ opacity: isDragging ? 0.5 : 1 }}>
{/* Your component's content */}
</div>
)
);
}
}
export default DropTarget('ITEM_TYPE', dropTargetSpec, dropTargetCollect)(
DragSource('ITEM_TYPE', dragSourceSpec, dragSourceCollect)(MyComponent)
);
A brief introduction to React DnD
React DnD (React Drag and Drop) is a popular library for implementing drag and drop functionality in React applications. It provides a set of high-level APIs for connecting DragSources and DropTargets to a DnD backend, such as HTML5 Drag and Drop API or a custom one. React DnD abstracts away the details of implementing drag and drop and makes it easier for developers to add this type of interaction to their applications.
React DnD works by defining the behavior of DragSources and DropTargets using higher-order components. DragSources describe what can be dragged, and DropTargets describe where things can be dropped. A DnD backend is responsible for tracking the state of the drag operation, such as the position of the drag source and the current drop target. React DnD also provides a store for storing the state of the drag operation, making it easier to persist the state across multiple components and even across multiple render cycles. The store is updated using actions that are dispatched from the DragSources and DropTargets, allowing developers to add custom logic for handling drag operations.
Most popular use cases for React DnD
- Implementing Drag and Drop Interactions: React DnD can be used to implement drag and drop interactions in React applications. This can be achieved by connecting DragSources and DropTargets to a DnD backend. For example, to define a DragSource, you can use the
useDrag
hook from React DnD, like so:
import { useDrag } from 'react-dnd';
const Item = ({ item }) => {
const [{ isDragging }, drag] = useDrag({
item,
collect: (monitor) => ({
isDragging: monitor.isDragging(),
}),
});
return (
<div ref={drag} style={{ opacity: isDragging ? 0.5 : 1 }}>
Drag Me
</div>
);
};
- Enabling Reordering of Elements: React DnD can be used to enable reordering of elements within a list. This can be achieved by connecting DragSources and DropTargets to a DnD backend, and using the state stored in the DnD store to keep track of the current order of the elements.
- Implementing Custom Drag and Drop Behaviors: React DnD allows developers to implement custom drag and drop behaviors by connecting DragSources and DropTargets to a custom DnD backend. This makes it possible to extend the functionality of the library to meet specific requirements for a particular use case.
It’s Really not that Complicated.
You can actually understand what’s going on inside your live applications.