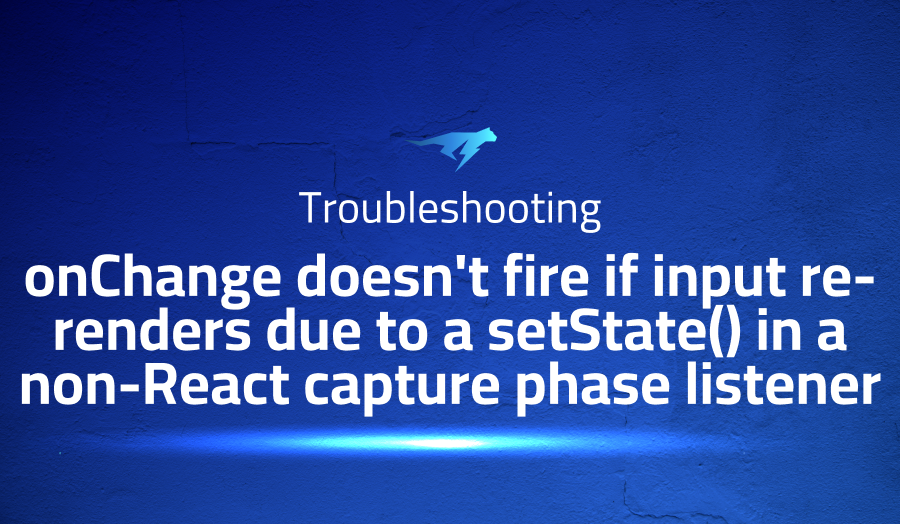
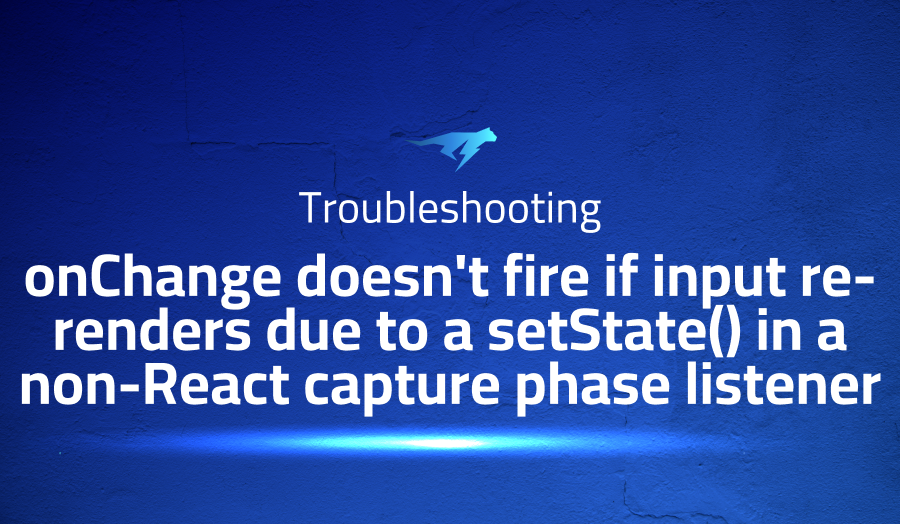
onChange doesn’t fire if input re-renders due to a setState() in a non-React capture phase listener
Explanation of the problem
The following issue has been present in React since its inception and can be reproduced up to React 0.11. Although this issue is rare and does not hold much practical significance, it is being reported for posterity purposes.
A minimal example that demonstrates the issue involves a React component, ‘App’, which includes an input element and a state object with a value property. The handleChange function updates the state object with the value entered into the input element. componentDidMount method attaches a listener to the document object that listens for ‘input’ events in the capture phase. When an ‘input’ event is detected, setState method is called, which leads to the issue.
The issue arises because the first setState call inside the capture phase listener function runs before the ChangeEventPlugin can decide whether to emit a change event. During this initial setState call, the input props still contain the old value (“”) while the DOM node’s value is new (“a”). Since these values are not equal, the DOM node value is set to “” (according to the props), and “” is remembered as the current value. Subsequently, when ChangeEventPlugin tries to determine whether to emit a change event, the tracker compares the newly set node.value (which is “”) with the lastValue stored by the tracker (which is also “”). As there are no changes, our “a” update is lost, and we never get the change event to set the correct state.
Troubleshooting with the Lightrun Developer Observability Platform
Getting a sense of what’s actually happening inside a live application is a frustrating experience, one that relies mostly on querying and observing whatever logs were written during development.
Lightrun is a Developer Observability Platform, allowing developers to add telemetry to live applications in real-time, on-demand, and right from the IDE.
- Instantly add logs to, set metrics in, and take snapshots of live applications
- Insights delivered straight to your IDE or CLI
- Works where you do: dev, QA, staging, CI/CD, and production
Start for free today
Problem solution for onChange doesn’t fire if input re-renders due to a setState() in a non-React capture phase listener
The issue at hand is related to conflicts between document event listeners and input onChange events in React applications. When both are used together, it is possible for the document event listener to interfere with the input onChange event, resulting in unexpected behavior. In particular, the input’s value may be updated in the DOM, but the state of the React component may not be updated, leading to inconsistencies.
One proposed solution is to use a lambda function to pass the event value to the handleChange method, instead of using it directly as an argument. This approach helps to avoid conflicts between document event listeners and input onChange events by ensuring that the event value is passed explicitly to the method. This way, the React state can be updated correctly, even if the DOM value is updated by a document event listener.
Another solution is to refactor the code to avoid using document event listeners altogether. This can be achieved by using React-specific event listeners instead of relying on document event listeners. For example, instead of using document.addEventListener, one can use the useEffect hook provided by React to attach event listeners to the component. This approach ensures that the event listeners are managed by React and are not interfering with the component’s state or lifecycle methods.
In summary, conflicts between document event listeners and input onChange events can lead to unexpected behavior in React applications. To avoid such issues, it is recommended to use a lambda function to pass the event value explicitly to the handleChange method or to refactor the code to use React-specific event listeners instead of relying on document event listeners. By following these best practices, React developers can ensure that their applications are robust and behave predictably in all scenarios.
Other popular problems with react
Problem: Performance Issues with Large Data Sets:
React applications can suffer from performance issues when rendering large data sets, such as tables or lists. The problem arises when React re-renders the entire component hierarchy each time a small change is made to the data set, even if the change affects only a small portion of the UI. This can result in slow rendering times, poor user experience, and unresponsive applications.
Solution:
One solution to this problem is to use pagination or virtualization techniques to reduce the amount of data being rendered at once. By splitting the data into smaller chunks or rendering only the visible portion of the data, React can achieve better performance and faster rendering times. Another solution is to use React’s memoization capabilities to avoid re-rendering the components that haven’t changed. This can be done by wrapping the components in the React.memo higher-order component or implementing the shouldComponentUpdate lifecycle method.
Problem: State Management
Managing state in complex React applications can be challenging and error-prone. As the number of components grows, it can become difficult to pass the state down the component hierarchy using props. Additionally, it can be challenging to keep track of the state changes and ensure that the correct components are re-rendered when the state changes.
Solution:
One popular solution to this problem is to use state management libraries, such as Redux or MobX. These libraries provide a centralized store for managing the state, making it easier to share state between components and keep track of state changes. Another solution is to use React’s built-in context API to pass the state down the component hierarchy without using props. This approach can be particularly useful when the state needs to be accessed by multiple components at different levels of the hierarchy.
Problem: Component Reusability
One of the main benefits of React is the ability to create reusable components. However, achieving true component reusability can be challenging, particularly when dealing with complex components that have multiple use cases. It can be difficult to design components that are flexible enough to be reused in different contexts without sacrificing their functionality.
Solution:
One solution to this problem is to use higher-order components (HOCs) or render props to create reusable components that can be customized for different use cases. HOCs are functions that take a component as an argument and return a new component with additional functionality. Render props, on the other hand, are functions passed as props to the component that provide the component with the necessary functionality. Another solution is to use component composition to create complex components by combining smaller, more focused components. This approach can help to keep the components simple and easy to reuse in different contexts.
A brief introduction of react
React is a JavaScript library for building user interfaces. It was developed by Facebook and is widely used in web development due to its efficient rendering, component-based architecture, and declarative programming approach. React is based on a virtual DOM (Document Object Model) which is a lightweight copy of the actual DOM. This allows React to update the UI in an efficient way by minimizing the number of manipulations needed to update the DOM. React provides a set of features and APIs to create reusable components which can be composed together to build complex UIs.
One of the key features of React is its use of a declarative programming approach. Instead of describing how the UI should change in response to user actions, developers simply declare the desired state of the UI, and React handles the rest. React provides a set of lifecycle methods which allow developers to hook into the component lifecycle and perform actions such as fetching data or setting up event listeners. React also supports server-side rendering which allows the initial render of the application to be done on the server before being sent to the client, improving performance and accessibility. React can be used with other libraries and frameworks, such as Redux for state management or Next.js for server-side rendering and routing.
In summary, React is a popular JavaScript library for building user interfaces with an efficient rendering system, component-based architecture, and declarative programming approach. React’s virtual DOM and lifecycle methods enable developers to create reusable components which can be composed together to build complex UIs. React supports server-side rendering, and can be used with other libraries and frameworks to enhance its functionality.
Most popular use cases for react
1. Building User Interfaces: React is primarily used for building user interfaces. React provides a set of features and APIs to create reusable components which can be composed together to build complex UIs. React components are self-contained modules of code that render a part of the UI based on their input props and state. Here is an example of a simple React component:
import React from 'react';
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
export default Greeting;
2. Single Page Applications: React can also be used to build single-page applications (SPAs) which provide a seamless user experience by loading content dynamically without requiring a full page reload. React can be used with other libraries and frameworks, such as React Router for client-side routing, to build SPAs. Here is an example of a simple SPA built using React:
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter, Route, Switch } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
const App = () => {
return (
<BrowserRouter>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</BrowserRouter>
);
};
ReactDOM.render(<App />, document.getElementById('root'));
3. Mobile and Desktop Applications: React can be used with React Native to build mobile applications for iOS and Android, and with Electron to build desktop applications for Windows, macOS, and Linux. React Native provides a set of components and APIs that map to the native UI elements of the target platform, allowing developers to build high-performance mobile apps with a single codebase. Electron allows developers to build cross-platform desktop apps using web technologies such as HTML, CSS, and JavaScript. Here is an example of a simple Electron app built using React:
import React from 'react';
import { render } from 'react-dom';
import App from './components/App';
render(<App />, document.getElementById('root'));
It’s Really not that Complicated.
You can actually understand what’s going on inside your live applications.